ControlAltDieliet

- Home /
- Mattermost /
- Introduction to Integrations /
- Mattermost API
Sending and receiving data with the Mattermost API
In the previous articles you learned how to send and request data with outgoing webhook, incoming webhooks and slash commands. In this article you learn how to do this with the Mattermost API.
The Mattermost API is the most powerfull way to have interactions between Mattermost and external software
Setting up webhooks or slash commands in Mattermost is very easy. Using the Mattermost API takes some more steps. But the API is way much more versatile and powerfull. Once you have made your first connection to the API, you will love it!
1 Create an Personal Access Token
You can work without a Personal Access Token and login with a username and password. You'll receive a temporary access token that will expire. Personal Acces Tokens do not expire are more secure because you don't have to store username and password in your code and using a personal access token gives you also full access to the API.
1.1 Go to the Menu.
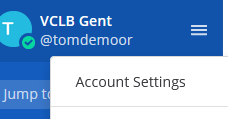
1.2 Go to Account Settings.
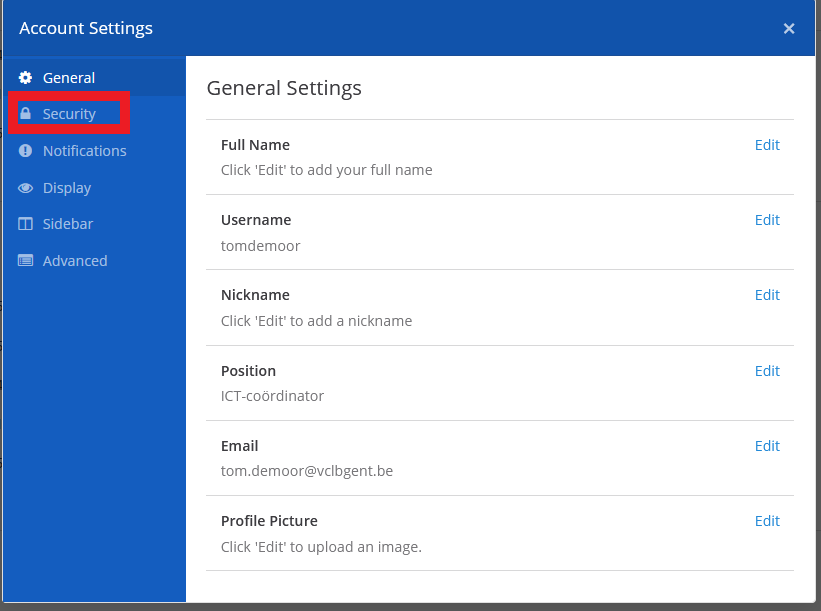
1.3 Go to Security, click at Edit to manage your Access Tokens.
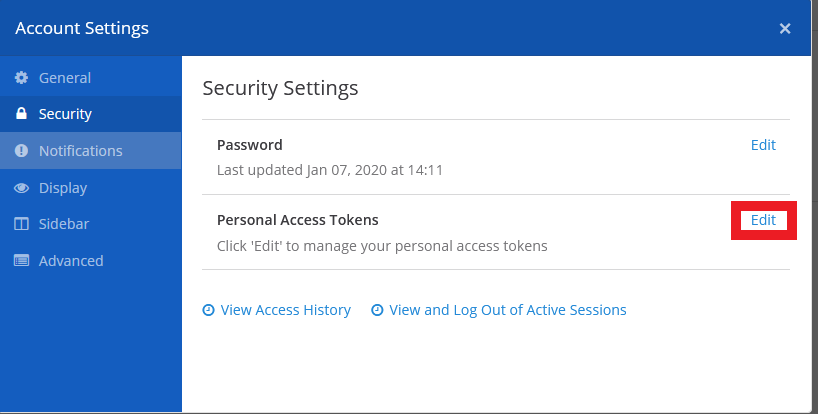
1.4 Now we create the Access Token in the next screen. Give a description for your Personal Access Token.

1.5 If you are generating an Personal Access Token with sysadmin rights, you'll receive a warning.
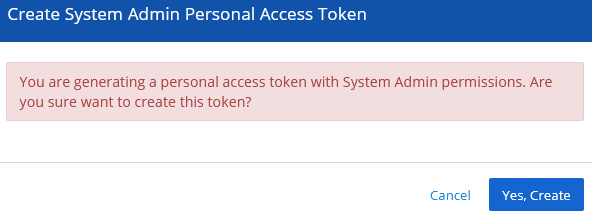
1.6 Your token is created. Do copy the Access Token! You can not recover it.
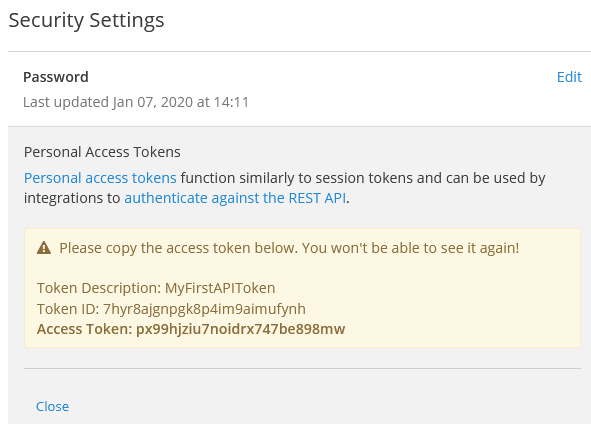
1.7 To be absolutely sure that you have copied the Access Token, you get an extra warning. Please ensure that you copied the token.
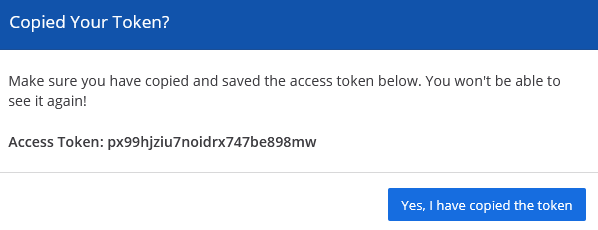
2 Write some code
OK, you did all what you needed to do in Mattermost to gain access to the Mattermost API.
In this blog you'll learn to use the API in Python. We'll be using the Mattermost Driver.
There are also drivers availble for JavaScript, Go and PHP.
2.1 First things first, installing the dependencies.
pip install mattermostdriver
2.2 Now let's build some magical Python code
First we import the necessary classes
from mattermostdriver import Driver
import json
2.3 Next step: Connecting to Mattermost
from mattermostdriver import Driver
import json
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"s1ern7edy3do9ggg9zdhakzyaw", ## THE TOKEN THAT YOU JUST CREATED
'scheme': 'https',
'port': 443
})
mm.login()
2.4 Let's write something to a channel!
You have to fill in two variables. Your teamname and the channel name. If you have spaces or special characters in your channel name, you can find the channel name by going to the channel in your browsers. You'll see the channel name in the url.
from mattermostdriver import Driver
import json
channel_name="YOUR-CHANNEL-NAME"
team="YOUR-TEAMNAME"
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"THE TOKEN THAT YOU JUST CREATED",
'scheme': 'https',
'port': 443
})
mm.login()
channel=mm.channels.get_channel_by_name_and_team_name(team, channel_name)
channel_id=channel['id']
mm.posts.create_post(options={
'channel_id': channel_id,
'message': 'This is a test through the API'
})
2.5 Listing to events
For listening to Mattermost you connect to a websocket. The events are passed to an event listener. In the example provided you will print all the events that are occuring. You receive the events in JSON.
from mattermostdriver import Driver
import json
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"THE TOKEN THAT YOU JUST CREATED",
'scheme': 'https',
'port': 443
})
mm.login()
async def my_event_handler(e):
message=json.loads(e)
print(message)
mm.init_websocket(my_event_handler)
3 More information
You can learn alot by watching the output that the event handler is printing.
Mattermost is providing great information on the API at https://api.mattermost.com/. You'll see you can do almost anything through the API.
The Python driver is well documentated too! You can read it on https://vaelor.github.io/python-mattermost-driver/.